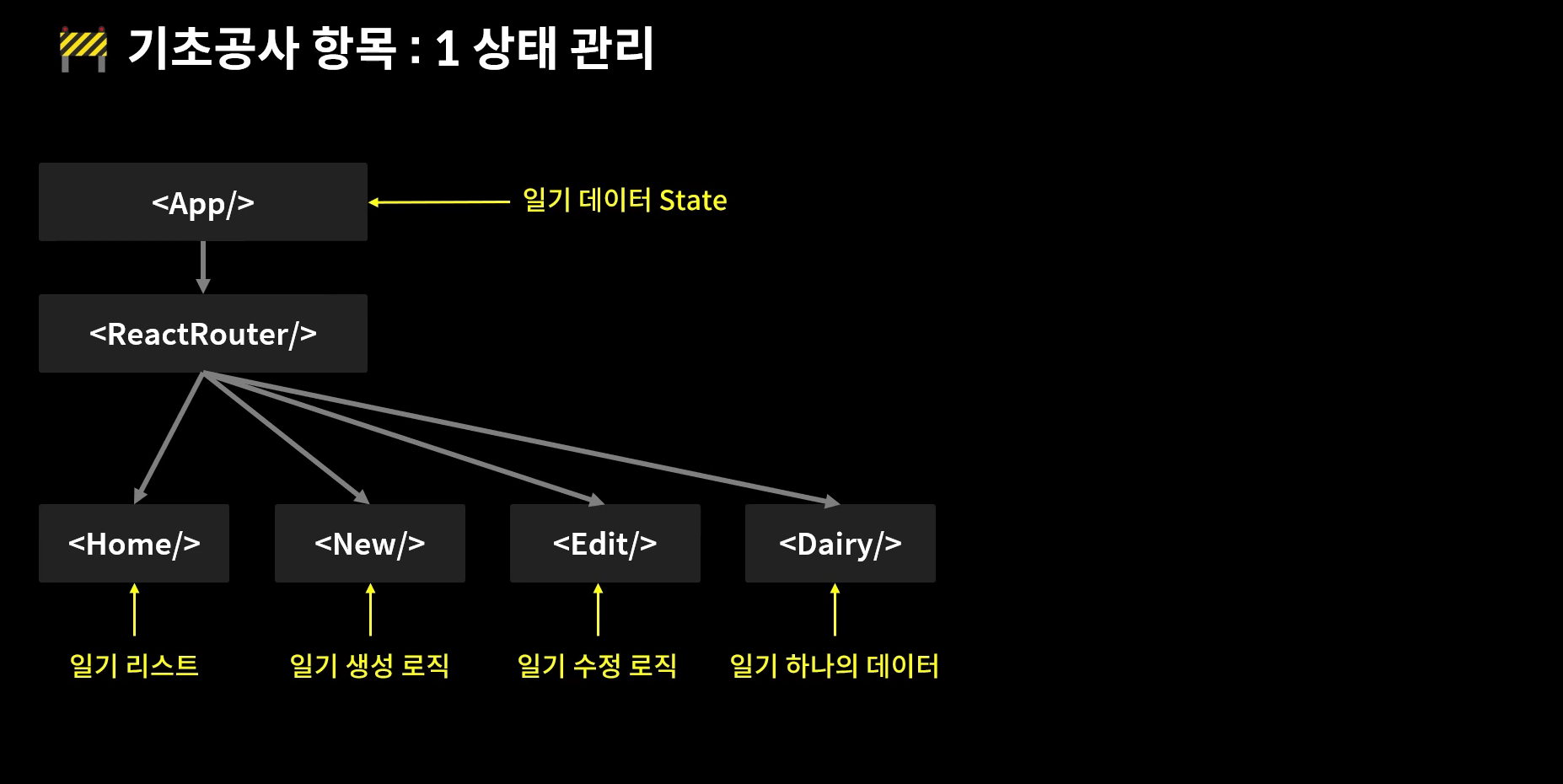
React Redux
useReducer();
상태관리 함수인 useState 대신 useReducer를 사용할 것이다.
컴포넌트 상태 업데이트 로직을 컴포넌트 바깥이나 다른파일에 작성 후 불러올 수 있다.
const [data, dispatch] = useReducer(reducer, []);
앱 컴포넌트안에 useReducer() 훅을 불러온다.
reducer
const reducer = (state, action) => {
let newState = [];
switch (action.type) {
case 'INIT': {
return action.data;
}
case 'CREATE': {
const newItem = {
...action.data
}
newState = [newItem, ...state];
break;
}
case 'REMOVE': {
newState = state.filter((it) => it.id !== action.data);
break;
}
case 'EDIT': {
newState = state.map((it) => it.id === action.data.id ? { ...action.data } : it);
break;
}
default:
return state;
}
return newState;
}
그리고 바깥쪽에 reducer 함수 작성
이제 dispatch 함수작성
dispatch
const [data, dispatch] = useReducer(reducer, []);
const dataId = useRef(0);
const onCreate = (date, content, emotion) => {
dispatch({
type: "CREATE", data: {
id: dataId.current,
data: new Date(date).getTime(),
content,
emotion
}
});
dataId.current += 1;
}
const onRemove = (targetId) => {
dispatch({ type: "REMOVE", targetId })
}
const onEdit = (targetId, date, content, emotion) => {
dispatch({
type: "EDIT",
data: {
id: targetId,
date: new Date(date).getTime(),
content,
emotion
}
})
}
contextProvider
[React] 컴포넌트 트리에 데이터 공급. useContext와 Provider
contextAPI를 다룰것이다 전달만 하는 비효율적인 props (프롭스드릴링)의 문제점을 해결할 것이다 모든 데이터를 가진 component가 provider 컴포넌트에게 모든 데이터를 준다. 그리고 공급자인 provider
h-owo-ld.tistory.com
컴포넌트 트리 전역을 감싸는 컨텍스트 프로바이더를 생성해주자
Context – React
A JavaScript library for building user interfaces
ko.reactjs.org
export const DiaryStateContext = React.createContext();
value={data}로 데이터를 공급해주었다
export const DiaryDispatchContext = React.createContext();
return (
<DiaryStateContext.Provider value={data}>
<DiaryDispatchContext.Provider value={(
onCreate,
onEdit,
onRemove
)}>
<BrowserRouter>
<div className="App">
<Routes>
<Route path="/" element={<Home />} />
<Route path="/new" element={<New />} />
<Route path="/edit" element={<Edit />} />
<Route path="/diary/:id" element={<Diary />} />
</Routes>
</div>
</BrowserRouter>
</DiaryDispatchContext.Provider>
</DiaryStateContext.Provider>
);
참고 :
- 한 입 크기로 잘라먹는 리액트
반응형
'개발 > Inafolio' 카테고리의 다른 글
[React] 미니프로젝트 - 일기장 글 작성/수정 화면 구현하기 (0) | 2022.08.09 |
---|---|
[React] 미니프로젝트 - 일기장 Home 화면 구현하기 (0) | 2022.08.05 |
[React] 프로젝트 기초공사하기 - 공통 컴포넌트, 폰트, 레이아웃 css 셋팅 (0) | 2022.08.02 |
[혼공단 8기] 4주차 미션 (0) | 2022.07.31 |
[혼공단 8기] 3주차 미션 (0) | 2022.07.24 |