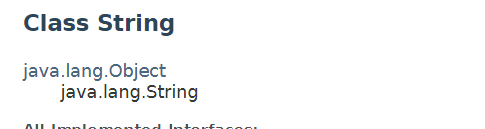
클래스 | 용도 | |
Object | - 자바 클래스의 최상위 클래스 | |
System | - 표준 입력 장치(키보드)로부터 데이터 입력받을 때 사용 - 표준 출력 장치(모니터)로부터 데이터 출력 시 사용 - 자바 가상 기계(JVM)를 종료할 때 사용 - 쓰레기 수집기(Garbage Collection)를 실행 요청할 때 사용 |
|
Class | - 클래스를 메모리로 로딩 시 사용 | |
String | - 문자열을 저장하고 여러 가지 정보를 얻을 때 사용 | |
Wrapper | Byte, Short, Character, Integer, Float, Double, Boolean, Long |
- 기본 타입의 데이터를 갖는 객체를 만들 때 사용 - 문자열을 기본 타입으로 변환 시 사용 - 입력값 검사에 사용 |
Math | - 수학 함수를 이용할 때 사용 |
API도큐먼트를 읽어보면서 책에서 다루지 않는 기능들도 습득하는걸 추천하셨다
Overview (Java SE 11 & JDK 11 )
This document is divided into two sections: Java SE The Java Platform, Standard Edition (Java SE) APIs define the core Java platform for general-purpose computing. These APIs are in modules whose names start with java. JDK The Java Development Kit (JDK) AP
docs.oracle.com
자바 API 도큐먼트?
API(Application Programming Interface) ; 라이브러리라고도 부르며, 프로그램 개발에 자주 사용되는 클래스 및 인터페이스의 모음을 뜻함
String, System 클래스들도 모두 API 속하는 클래스이다.
Object 클래스
클래스 선언시 extends 키워드로 다른 클래스를 상속하지 않더라도 암시적으로는 java.lang.Object클래스를 상속받는다.
Object는 자바의 최상위 부모 클래스에 해당한다.
Object (Java SE 11 & JDK 11 )
Called by the garbage collector on an object when garbage collection determines that there are no more references to the object. A subclass overrides the finalize method to dispose of system resources or to perform other cleanup. The general contract of fi
docs.oracle.com
Object 클래스에 있는 equals() 메소드 예시
두 객체를 동등 비교할 때 equals()를 주로 사용 >> 객체가 저장하는 데이터가 동일함을 뜻함
public class Member {
public String id;
public Member(String id) {
super();
this.id = id;
}
@Override
public boolean equals(Object obj) {
if(obj instanceof Member) {
Member member = (Member) obj;
if (id.equals(member.id)) {
return true;
}
}
return false;
}
}
public class MemberEx {
public static void main(String[] args) {
Member obj1 = new Member("blue");
Member obj2 = new Member("blue");
Member obj3 = new Member("red");
if (obj1.equals(obj2)) {
System.out.println("obj1과 obj2는 동등합니다");
} else {
System.out.println("obj1과 obj2는 동등하지 않습니다");
}
if (obj1.equals(obj3)) {
System.out.println("obj1과 obj3는 동등합니다");
} else {
System.out.println("obj1과 obj3는 동등하지 않습니다");
}
}
}
객체 해시코드(hashCode())
객체를 식별하는 하나의 정수값. Object클래스의 hashCode()메소드는 객체의 메모리 번지를 이용해서 해시코드를 만들어 리턴하기 때문에 객체마다 다른값을 가진다.
논리적 동등비교시 hashCode()를 오버라이딩 할 필요가 있는데, 컬렉션 프레임워크에서 HashSet, HashMap, Hashtable은 hashCode()메소드를 실행해서 리턴된 해시코드값이 같은지를 보고, 같으면 equals()메소드로 다시 비교한다. 따라서 해시코드메소드가 true로 나와도, 이퀄스 리턴값이 다르면 다른객체가 된다
public class Key {
public int number;
public Key(int number) {
super();
this.number = number;
}
@Override
public boolean equals(Object obj) {
if(obj instanceof Key) {
Key compareKey = (Key) obj;
if (this.number == compareKey.number) {
return true;
}
}
return false;
}
}
public class KeyEx {
public static void main(String[] args) {
//Key 객체를 식별키로 사용해서 String값을 저장하는 HashMap 객체 생성
HashMap<Key, String> hashMap = new HashMap<Key, String>();
//식별키 new Key(1)로 "홍길동"을 저장함
hashMap.put(new Key(1), "홍길동");
//식별키 new Key(1)로 "홍길동"을 읽어옴
String value = hashMap.get(new Key(1));
System.out.println(value);
}
}
Key 클래스에 hashCode()의 메소드또한 재정의 해줘서 논리적으로 동등한 객체일 경우 동일한 해시코드가 리턴되도록 해야한다
System.nanoTime();
public class SystemTimeEx {
public static void main(String[] args) {
long time1 = System.nanoTime();
long time2 = System.currentTimeMillis();
int sum = 0;
for (int i = 0; i <= 1000000; i++) {
sum += i;
}
long time3 = System.nanoTime();
long time4 = System.currentTimeMillis();
System.out.println("1~1000000까지의 합: " + sum);
System.out.println("계산에 " + (time3 - time1) + "nano초가 소요되었습니다");
System.out.println("계산에 " + (time4 - time2) + "ms초가 소요되었습니다");
}
}
Class 객체정보, 절대경로 얻기
public class ClassEx {
public static void main(String[] args) throws Exception {
Class clazz = Car.class;
System.out.println(clazz.getName());
System.out.println(clazz.getSimpleName());
System.out.println(clazz.getPackage().getName());
System.out.println(clazz.getPackageName());
System.out.println(clazz.getResource("photo1.jpg").getPath());
}
}
String 클래스
public class ByteTostringEx {
public static void main(String[] args) {
byte[] bs = {72, 101, 108, 108, 101, 32, 74, 97, 118, 97 };
String str1 = new String(bs);
System.out.println(str1);
String str2 = new String(bs, 6, 4);
System.out.println(str2);
}
}
public class KeyToStringEx {
public static void main(String[] args) throws IOException {
byte[] bs = new byte[100];
System.out.println("입력: ");
int readByNo = System.in.read(bs);
String str = new String(bs, 0, readByNo - 2);
System.out.println(str);
}
}
이외에도 charAt(); (인덱스 문자 리턴), indexOf(), length(), replace() 많이사용
public class CharAtEx {
public static void main(String[] args) {
String ssn = "010624-1230123";
char sex = ssn.charAt(7);
switch (sex) {
case '1': case '3':
System.out.println("남자");
break;
case '2': case '4':
System.out.println("여자");
break;
}
}
}
public class IndexOfEx {
public static void main(String[] args) {
String subject = "자바 프로그래밍";
int location = subject.indexOf("프로그래밍");
System.out.println(location);
if (subject.indexOf("자바") != -1) {
System.out.println("자바와 관련된 책이군요");
} else {
System.out.println("자바와 관련없는 책이군요");
}
}
}
public class LengthEx {
public static void main(String[] args) {
String ssn = "73062412301123";
int length = ssn.length();
if (length == 13) {
System.out.println("주민번호 확인");
} else {
System.out.println("주민번호 자릿수 오류");
}
}
}
public class ReplaceEx {
public static void main(String[] args) {
String oldString = "자바는 객체 지향입니다, 자바는 풍부한 API를 지원합니다";
String newString = oldString.replace("자바", "Java");
System.out.println(oldString);
System.out.println(newString);
}
}
public class LowerUpperEx {
public static void main(String[] args) {
String str1 = "Java Programming";
System.out.println(str1);
System.out.println(str1.toLowerCase());
System.out.println(str1.toUpperCase());
}
}
public class TrimEx {
public static void main(String[] args) {
String tel1 = " 02";
String tel2 = " 123 ";
String tel3 = " 8945 ";
String tel = tel1.trim()+"-"+tel2.trim()+"-"+tel3.trim();
System.out.println(tel);
}
}
Wrapper클래스
기본타입의 값을 갖는 포장객체를 생성(Boxing) >> 기본타입 값을 내부에 두고 포장하여 외부에서 변경할 수 없게 한다.
만약 내부의 값을 변경하고 싶다면 새로운 포장(wrapper)객체를 만들어야한다
기본타입 | 포장클래스 |
byte | Byte |
char | Character |
short | Short |
int | Integer |
long | Long |
float | Float |
double | Double |
boolean | Boolean |
Boxing된 포장객체에서 다시 기본타입의 값을 얻기위해서는(Unboxing) 기본타입이름 + Value()메서드 활용
포장값 비교시에는 equals() 사용해야함 (메소드로 내부값 비교)
public class BoxingUnboxing {
public static void main(String[] args) {
//박싱
Integer obj1 = new Integer(100);
Integer obj2 = new Integer("200");
Integer obj3 = Integer.valueOf(300);
//언박싱
int value1 = obj1.intValue();
int value2 = obj2.intValue();
int value3 = obj3.intValue();
System.out.println(value1);
System.out.println(value2);
System.out.println(value3);
//자동박싱
Integer obj = 100;
System.out.println("value: " + obj.intValue());
//대입 시 자동 언박싱 : 포장클래스 타입에 기본값이 대입될 경우
int value = obj;
System.out.println("value: " + value);
//연산 시 자동 언박싱
int result = obj + 100;
System.out.println("result: " + result);
}
}
Math 클래스
메소드 | 설명 |
Math.abs() | 절대값 |
Math.ceil() | 올림값 |
Math.floor() | 버림값 |
Math.max() | 최대값 |
Math.min() | 최소값 |
Math.random() | 랜덤값 |
Math.rint() | 가까운 정수의 실수값 |
Math.rount() | 반올림값 |
public class MathEx {
public static void main(String[] args) {
int num = (int)(Math.random() * 6) + 1;
System.out.println("주사위 눈 : " + num);
}
}
정리
Object 클래스 : 자바의 최상위 부모 클래스로 모든 자바객체에서 사용 가능
System 클래스 : 프로그램 종료, 입출력 등 운영체제의 일부 기능 사용. System 클래스의 모든 필드와 메소드는 정적필드와 정적메소드로 구분되어있다
Class 클래스 : 클래스와 인터페이스의 메타데이터(클래스이름, 생성자정보, 필드정보, 메소드정보) 관리.
String 클래스 : 문자열 조작과 관련된 메소드
Wrapper 클래스 : 기본타입의 값을 기본타입의 값을 갖는 객체(포장객체)로 만들기도 하고(박싱), 포장객체로부터 기본타입의 값을 얻기도한다(언박싱)
Math 클래스 : 수학 계산에 사용할 수 있는 메소드 제공. 모두 정적메소드이기 때문에 Math클래스로 바로 사용 가능
참고 :
- 혼자 공부하는 자바(신용권), 한빛미디어
'개발 > JAVA' 카테고리의 다른 글
[Java] 멀티 스레드 1 (0) | 2022.07.25 |
---|---|
[Java] java.util 패키지 내 Date, Calendar 클래스 (0) | 2022.07.21 |
[Java] 예외처리 (0) | 2022.07.18 |
[Java] 익명 객체 (0) | 2022.07.13 |
[Java] 중첩클래스와 중첩 인터페이스 (0) | 2022.07.13 |